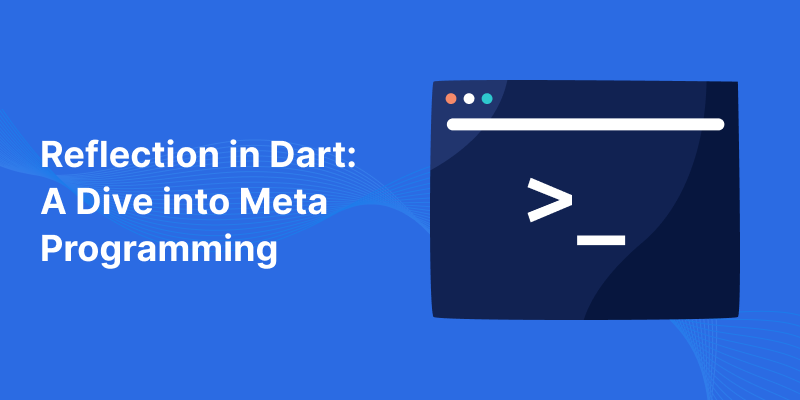
Have you ever wondered how programming languages enable you to inspect and modify their own structure at runtime? Well, if you’re familiar with Dart, you might have encountered the concept of reflection.
Reflection is a powerful feature that allows Dart programs to introspect their own structure, types, and metadata at runtime. In this blog post, we’ll take a closer look at reflection in Dart, exploring its uses, advantages, and some practical examples.
Before we dive into the blog, you can also check our Flutter app development company page.
Understanding Reflection
Reflection is the ability of a program to examine its own structure, types, and metadata at runtime. In Dart, reflection is made possible through the dart:mirrors
library. This library provides classes and functions that allow you to inspect and manipulate the structure of Dart objects, classes, libraries, and functions dynamically.
Use Cases for Reflection
Reflection in Dart opens up a wide range of possibilities for metaprogramming, dynamic code generation, and runtime analysis. Some common use cases for reflection include:
- Serialization and Deserialization: Reflection can be used to automatically convert Dart objects to and from JSON representations, without the need for manual mapping.
- Dependency Injection: Reflection allows frameworks like AngularDart to automatically inject dependencies into classes at runtime based on type annotations.
- Object Inspection: You can use reflection to examine the properties, methods, and metadata of Dart objects dynamically, enabling tasks such as debugging and testing.
- Dynamic Code Generation: Reflection enables the creation of code generators and builders that can generate Dart code dynamically based on runtime information.
Advantages and Disadvantages
While reflection provides powerful capabilities, it also comes with some trade-offs:
Advantages:
- Flexibility: Reflection allows you to write more dynamic and flexible code that can adapt to changing requirements at runtime.
- Reduced Boilerplate: By leveraging reflection, you can reduce the amount of boilerplate code needed for tasks like serialization, deserialization, and dependency injection.
- Metaprogramming: Reflection enables advanced metaprogramming techniques, allowing you to write code that can modify its own structure and behaviour dynamically.
Disadvantages:
- Performance Overhead: Reflection can incur a significant performance overhead compared to statically typed code, as it involves runtime introspection and dynamic method invocation.
- Code Obfuscation: Since reflection relies on runtime metadata, it can make code obfuscation techniques less effective, potentially exposing implementation details.
- Complexity: Reflection introduces additional complexity to your codebase, making it harder to reason about and maintain.
Practical Examples
Let’s explore some practical examples of reflection in Dart:
Serialization/Deserialization
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
import 'dart:convert'; import 'dart:mirrors'; class Serializable { Map<String, dynamic> toJson() { var json = <String, dynamic>{}; InstanceMirror instanceMirror = reflect(this); ClassMirror classMirror = instanceMirror.type; classMirror.declarations.forEach((symbol, declaration) { if (declaration is VariableMirror) { var key = MirrorSystem.getName(symbol); var value = instanceMirror.getField(symbol).reflectee; json[key] = value; } }); return json; } } class Person extends Serializable { String name; int age; Person(this.name, this.age); } void main() { var person = Person('John Doe', 30); var json = jsonEncode(person.toJson()); print(json); // Output: {"name":"John Doe","age":30} } |
Dependency Injection
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import 'dart:mirrors'; class Injectable { const Injectable(); } @Injectable() class Logger { void log(String message) { print(message); } } void main() { ClassMirror classMirror = reflectClass(Logger); Logger logger = classMirror.metadata.first.reflectee; logger.log('Hello, world!'); // Output: Hello, world! } |
Conclusion
Reflection in Dart is a powerful feature that enables metaprogramming, dynamic code generation, and runtime analysis. While it offers flexibility and reduced boilerplate, it also comes with performance overhead and increased complexity. By understanding its capabilities and limitations, you can leverage reflection to write more dynamic and flexible Dart applications. Whether you’re building a web application, a command-line tool, or a mobile app with Flutter, reflection can be a valuable tool in your Dart programming arsenal.
To learn more about the package please visit here.
Thanks for reading. You can also check other blogs from here. Happy coding.