Hi folks, today we will have a quick look at the Singleton Pattern in Swift. In this blog, we will also check how and why should we use Singleton on our code.
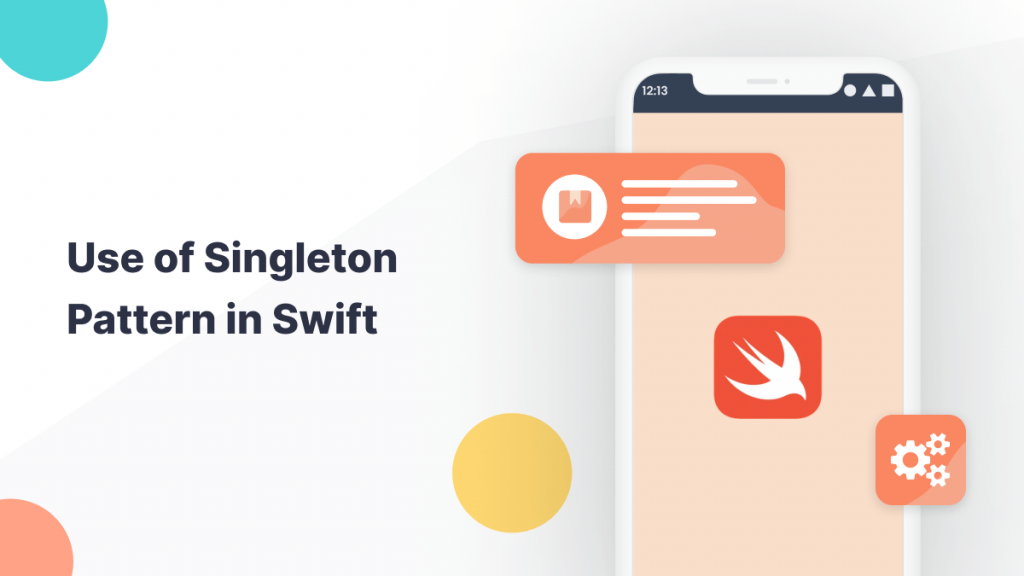
What is Singleton?
In Swift, a singleton is a design pattern that ensures that a class will have only one instance and provide a global point of access to that instance.
Singleton initializes your class instance only once with static property and it will share your class instance globally throughout the application.
your class instance globally throughout the application.
Before we dig deeper into the Singletone, check out our Flutter App Development Services.
Implementation of Singleton Pattern in Swift
Check out the step-by-step process for converting a class to a singleton class.
Step 1:- Create a Simple Class
Here we will create a class named SingletonTest and add some methods and properties to it.
1 2 3 4 5 6 7 8 9 |
class SingletonTest { init() { } let inputValue : Int = 10 func testFunction() { print("Executing test function of SingletonTest class") } } |
Step 2:- Mark the init method Private
1 2 3 4 5 6 7 8 9 10 |
class SingletonTest { private init() { } let inputValue : Int = 10 func testFunction() { print("Executing test function of SingletonTest class") } } |
By marking the init method of the class private, it will become inaccessible outside the class and will not allow any variable to create an instance of it. With this, we have successfully created a singleton class out of a regular class.
Step 3:- Access the Methods of the class
As we know, to access the method of any class we need to have an instance of it. Now since we cannot access our singleton class outside of its scope we need to initialize a class within the scope.
1 2 3 4 5 6 7 8 9 10 11 |
class SingletonTest { static let instance = SingletonTest() private init() { } let inputValue : Int = 10 func testFunction() { print("Executing test function of SingletonTest class") } } |
Once we created the instance of the class we can now access the methods and properties of the SingletonTest class by accessing the instance of the class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
class SingletonTest { static let instance = SingletonTest() private init() { } let inputValue : Int = 10 func testFunction() { print("Executing test function of SingletonTest class") } } var object = SingletonTest.instance print(object.inputValue) object.testFunction() /// Output:- 10 Executing test function of SingletonTest class |
Why and Where to Use Singleton
A basic idea behind using singleton classes is memory management. As it provides Global Access and by using a single instance for the class it doesn’t allocate separate memory for every reference.
Singleton classes can be used in various scenarios where we are using a class for a single common purpose like network calling, data storage and session management.
Conclusion
So, In this article, we have learned how can we implement singleton patterns in our classes and their use cases.
You can also check more informative blogs on Swift with Mobikul Blogs.