In Flutter development, understanding common exceptions is crucial for maintaining a smooth user experience and app functionality. This blog post on ‘Understanding Common Exceptions in Flutter’ will cover the most frequent exceptions, their causes, and best practices for handling them effectively.
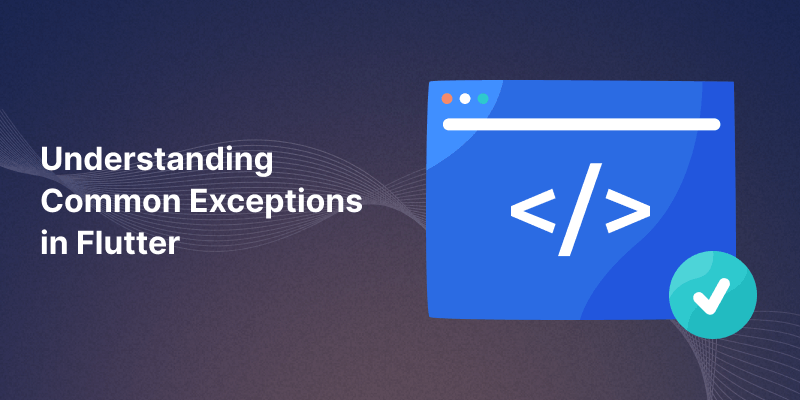
1. Null Pointer Exception
Description: This exception occurs when a null value is accessed, leading to runtime errors.
Causes:
- Improper initialization of variables.
- Attempting to access properties or methods on null objects.
Best Practices:
- Use null safety features in Dart.
- Implement null checks before accessing variables.
1 2 |
String? name; print(name.length); // Throws Null Pointer Exception |
2. Type Casting Exception
Description: This occurs when there’s an attempt to cast an object to a type it doesn’t belong to.
Causes:
- Incorrect data type assignments.
- Passing arguments of the wrong type to functions.
Best Practices:
- Validate types before casting.
- Use
is
checks for type compatibility.
1 2 |
dynamic value = 'Hello'; int number = value as int; // Throws Type Casting Exception |
3. Index Out-of-Range Exception
Description: This exception arises when trying to access an index that is outside the bounds of a list.
Causes:
- Accessing an index greater than or equal to the list length.
Best Practices:
- Always check the length of the list before accessing an index.
1 2 |
List<int> numbers = [1, 2, 3]; print(numbers[3]); // Throws Index Out of Range Exception |
4. Format Exception
Description: This occurs when parsing a string to a different type fails due to incorrect formatting.
Causes:
- Invalid string formats during parsing operations.
Best Practices:
- Validate string formats before parsing.
- Use try-catch blocks to handle potential exceptions.
Example:
1 2 |
String input = 'abc'; int number = int.parse(input); // Throws Format Exception |
5. State Error
Description: Triggered when a method is called at an inappropriate time, often after an object has been disposed of.
Causes:
- Calling methods on disposed objects.
Best Practices:
- Ensure that the object is still active before calling methods.
Example:
1 2 3 |
final controller = TextEditingController(); controller.dispose(); controller.text = 'Hello'; // Throws State Error |
6. Network Exception
Description: These exceptions include connection failures, timeouts, or server errors that affect network communication.
Causes:
- Poor internet connectivity.
- Server-side issues.
Best Practices:
- Implement retry logic for failed requests.
- Provide user feedback for network issues.
Example:
1 2 3 4 5 6 7 8 9 10 |
import 'dart:io'; void fetchData() async { try { // Simulated network request throw SocketException('No Internet'); } catch (e) { print('Network Exception: $e'); // Handles network exceptions } } |
You can also check the Flutter official site for more information about error and exception handling.
Understanding and handling exceptions in Flutter is crucial for developing robust applications. By implementing best practices and using proper error-handling techniques, developers can enhance the user experience and maintain app stability. Regularly reviewing and testing code can also help in identifying and resolving potential exceptions before they impact users.
Thanks for reading. You can also check other blogs from here. Happy coding.