Speech_To_Text In Flutter is used to convert voice or speech into the form of text or converting spoken words into written texts.
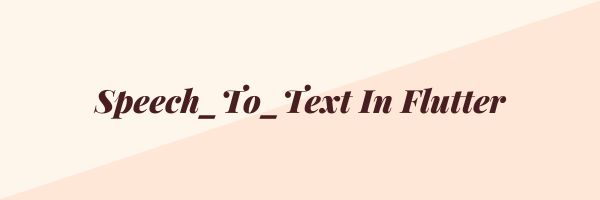
Before we start, have a look at flutter app development services from mobikul.
In this blog, we are using three packages to completely demonstrate the speech to text in a flutter.
- speech_to_text – It will recognize the speech or voice and then convert it into the respective text.
- highlight_text – It will be used to highlight the selected words which we initially give to it.
- avatar_glow – It will be used for the glow effect around the button. In this blog, we are using it to give the glow effect around the listen to speak button.
Here, we are also going to use the confidence feature which measures the degree of accuracy for the response.
Let’s Start The Steps For Integrating Speech_To_Text In Flutter
Step1: Create a flutter project.
Step2: Add the below dependencies in pubspec.yaml file.
1 2 3 |
speech_to_text: ^3.1.0 highlight_text: ^0.7.2 avatar_glow: ^1.2.0 |
In order to check the updated version of the dependency click here.
Step3: Now add permission for record voice in AndroidManifest.xml file.
1 2 3 |
<uses-permission android:name="android.permission.RECORD_AUDIO" /> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> |
Step4: We should make sure that minSdkVersion must be greater than 21.
1 |
minSdkVersion 21 |
Step5: Now start coding for the same.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 |
import 'package:flutter/material.dart'; import 'package:avatar_glow/avatar_glow.dart'; import 'package:highlight_text/highlight_text.dart'; import 'package:speech_to_text/speech_to_text.dart' as speechToText; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { speechToText.SpeechToText speech; String textString = "Press The Button"; bool isListen = false; double confidence = 1.0; final Map<String, HighlightedWord> highlightWords = { "flutter": HighlightedWord( textStyle: TextStyle(color: Colors.redAccent, fontWeight: FontWeight.bold)), "developer": HighlightedWord( textStyle: TextStyle(color: Colors.redAccent, fontWeight: FontWeight.bold)), }; void listen() async { if (!isListen) { bool avail = await speech.initialize(); if (avail) { setState(() { isListen = true; }); speech.listen(onResult: (value) { setState(() { textString = value.recognizedWords; if (value.hasConfidenceRating && value.confidence > 0) { confidence = value.confidence; } }); }); } } else { setState(() { isListen = false; }); speech.stop(); } } @override void initState() { super.initState(); speech = speechToText.SpeechToText(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Speech To Text"), ), body: Column( children: [ SizedBox( height: 10.0, ), Container( child: Text( "Confidence: ${(confidence * 100.0).toStringAsFixed(1)}%", style: TextStyle( fontSize: 20.0, fontWeight: FontWeight.bold, color: Colors.red), ), ), Container( padding: EdgeInsets.all(20), child: TextHighlight( text: textString, words: highlightWords, textStyle: TextStyle( fontSize: 25.0, color: Colors.black, fontWeight: FontWeight.bold), ), ) ], ), floatingActionButton: AvatarGlow( animate: isListen, glowColor: Colors.red, endRadius: 65.0, duration: Duration(milliseconds: 2000), repeatPauseDuration: Duration(milliseconds: 100), repeat: true, child: FloatingActionButton( child: Icon(isListen ? Icons.mic : Icons.mic_none), onPressed: () { listen(); }, ), ), ); } } |
Finally, we can run the code and as a result, we can see the below output.
Conclusion
In this blog, we have discussed Speech_To_Text In Flutter.
I hope it will help you out in understanding and getting a brief about it.
Similarly, for more interesting blogs check out here – https://mobikul.com/blog/
Thank you for reading!!
Reference
https://pub.dev/packages/speech_to_text