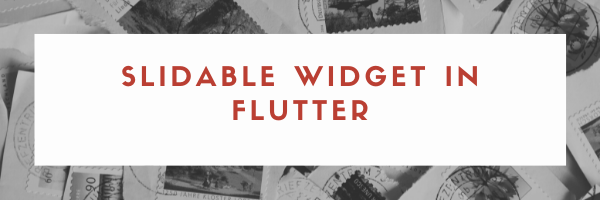
Hello everyone In this blog, we will learn and implement new interesting things in flutter. Like, here we will be using a Slidable Widget in flutter. In this blog, we will share the easiest and best way to implement a Slidable Widget in flutter using a plugin.
You may also check our interactive app designs by our Flutter app development company
A Slidable Widget in flutter is a widget that let us add actions on your list items when we are sliding it to the left or to the right.
Features of the widget
- we will be able to perform actions on dismissing the tile by using the dismissible property of the widget.
- we will also be able to add multiple slide actions by using the SlidableAction property of the widget.
- There are mainly four types of motion that we can give to the slide [ Behind Motion, Drawer Motion, Scroll Motion, Stretch Motion ].
Implementation:
Step1: Add the flutter_slidable dependency to pubspec.yaml file.
Step2: Run “flutter pub get” in the root directory of your app.
1 |
flutter_slidable: ^1.2.0 |
In order to check the updated version of the dependency click here.
Step3: Now start the coding for the same.
In this example, I am using DrawerMotion.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
import 'package:flutter/material.dart'; import 'package:flutter_slidable/flutter_slidable.dart'; class MySlider extends StatefulWidget { const MySlider({Key? key}) : super(key: key); @override _MySliderState createState() => _MySliderState(); } class _MySliderState extends State<MySlider> { List myList = ["India", "Nepal", "Sri Lanka", "America", "United Kingdom"]; void doNothing(BuildContext context) {} @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text("Slidable Widget In Flutter"), ), body: myList.isNotEmpty ? ListView.builder( itemCount: myList.length, itemBuilder: (context, index) { return Padding( padding: const EdgeInsets.all(8.0), child: Slidable( key: const ValueKey(0), endActionPane: ActionPane( dismissible: DismissiblePane(onDismissed: () { // we can able to perform to some action here }), motion: const DrawerMotion(), children: [ SlidableAction( autoClose: true, flex: 1, onPressed: (value) { myList.removeAt(index); setState(() {}); }, backgroundColor: Colors.red, foregroundColor: Colors.white, icon: Icons.delete, label: 'Delete', ), SlidableAction( autoClose: true, flex: 1, onPressed: (value) { myList.removeAt(index); setState(() {}); }, backgroundColor: Colors.blueAccent, foregroundColor: Colors.white, icon: Icons.edit, label: 'Edit', ), ], ), child: Container( color: Colors.yellow, width: MediaQuery.of(context).size.width, height: 50, child: Center( child: Text( myList[index], style: const TextStyle( color: Colors.black, fontWeight: FontWeight.bold, fontSize: 18), )), ), ), ); }, ) : const Center( child: Text("Your List is Empty", style: TextStyle( color: Colors.black, fontWeight: FontWeight.bold, fontSize: 18)), ), ); } } |
Output
Conclusion
Congratulations!! You have learned how to use Slidable Widget in flutter.
For more detail, you can refer to the official doc of a flutter here.
For more interesting blogs check out here – https://mobikul.com/blog/
Thanks for reading!!.