Hello readers, today we will learn how we can parse XML data into NSDictionary in Swift.
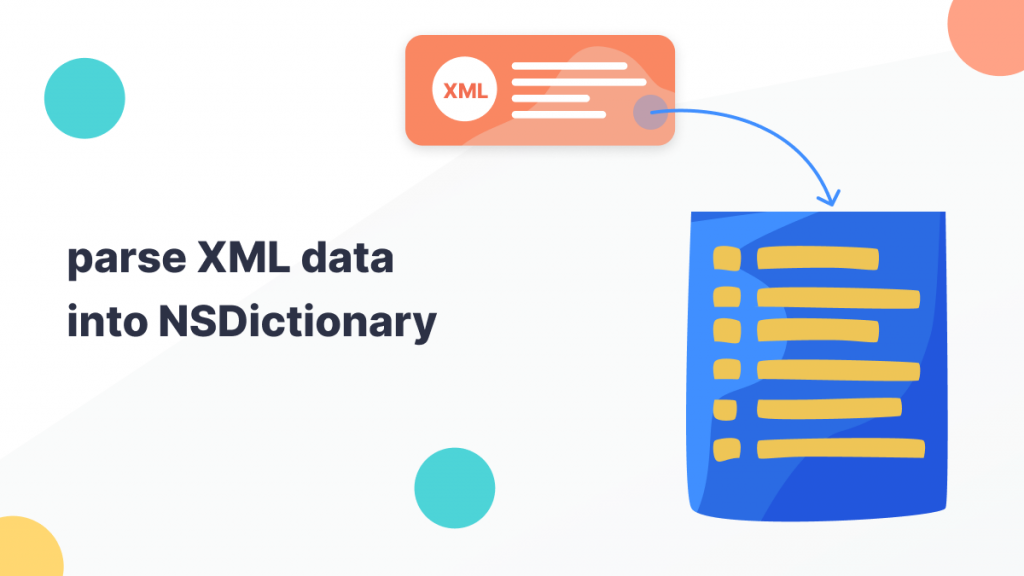
Introduction
XML (Extensible Markup Language) is a popular and widely used format for representing data in a structured manner. To use the XML data on our Applications and Programs we parse the data same as for the JSON and format it according to the needs of our code.
In Swift, there are many ways as well as libraries that can parse the XML data for our application. In this article, we will use XMLParser, the most commonly used and straightforward way of parsing XML data.
You can also learn about our Flutter Development Services here.
Parse XML data into NSDictionary
The NSDictionary in Swift is a collection type under the Foundation framework that stores the data in key-value pairs. You can store your XML data in NSDictionary by following the below steps.
Step:- 1. Import Foundation
Firstly, import the Foundation at the beginning of your swift file.
1 |
import Foundation |
Step:-2 Create a class and extend the XMLParsers delegates
After adding the Foundation, you need to create a class with XMLParserDelegate extended and add some variables to it.
1 2 3 4 5 |
class XMLParses : NSObject, XMLParserDelegate { var dictionary = NSMutableDictionary() var key : String? var value : String? } |
Now add the below methods one by one on the XMLParses class we created.
1 2 3 4 5 6 7 8 9 10 |
func parseXML(xmlData: Data) -> NSDictionary? { let parser = XMLParser(data: xmlData) parser.delegate = self if parser.parse() { return dictionary } else { print("Process Failed!") return nil } } |
The above method will be the main method that we will be calling for parsing the data and will return the dictionary with the parsed XML data.
1 2 3 4 5 6 7 8 |
func parser(_ parser: XMLParser, didStartElement elementName: String, namespaceURI: String?, qualifiedName qName: String?, attributes attributeDict: [String : String] = [:]) { key = elementName } func parser(_ parser: XMLParser, foundCharacters string: String) { value = string.trimmingCharacters(in: .whitespacesAndNewlines) } |
The above methods will set our key and value for the dictionary. Once the above method sets the key and value we need to call the below method to add those key values in the dictionary.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
func parser(_ parser: XMLParser, didEndElement elementName: String, namespaceURI: String?, qualifiedName qName: String?) { if let element = key, let value = value { if let existingValue = dictionary[element] as? String { dictionary[element] = [existingValue, value] }else if let existingValue = dictionary[element] as? [String] { dictionary[element] = existingValue + [value] }else{ dictionary[element] = value } } key = nil value = nil } |
Step:- 3 Call the Function with XML Data
Once the above setup is completed, you will have to call the parseXML method to initiate the parsing of the XML data.
1 2 3 4 5 6 7 8 |
let xmlString = "<company><firstname>Webkul</firstname><lastname>Mobikul</lastname></company>" if let xmlData = xmlString.data(using: .utf8){ let parseXML = XMLParses() if let responsedictionary = parseXML.parseXML(xmlData: xmlData){ print(responsedictionary) } } |
After calling the main method, you will see the output for the XML string data as:-
1 2 3 4 |
{ firstname = Webkul; lastname = Mobikul; } |
Conclusion
So, In this article, we have learned about how can we parse XML data into NSDictionary.
You can also learn more about Swift with Mobikul Blogs.