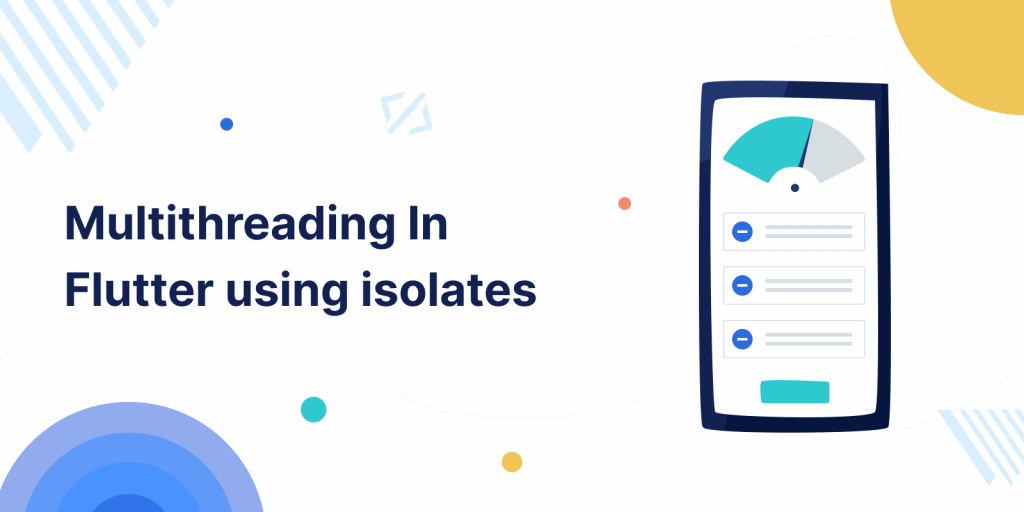
Thread is a lightweight process or small set of instructions that can be executed and scheduled by the CPU independently of the parent process. Multithreading refers to execute multiple threads at a time to achieve concurrency and multiprocessing in programming.
Dart is single thread language even when we are using asynchronous programming using async / await. To execute expensive operation such as accessing multiple API requests synchronously, Image processing, working with animations. Today we will implement multithreading in flutter using isolates.
Isolates allows to run the task without blocking the main thread, to provide the smooth and responsive user experience.
You can find out more about the Flutter app development services page.
Implementation
First create a stateless widget with simple user interface having circular progress indicator and elevated button as shown below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
class RepoScreen extends StatelessWidget { const RepoScreen({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text("Isolates exaple"), ), body: SizedBox( width: MediaQuery.of(context).size.width, child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ const Padding( padding: EdgeInsets.symmetric(vertical: 8.0), child: CircularProgressIndicator(), ), ElevatedButton(onPressed: () async { executeExpensiveOperation(); }, child: const Text("Execute operation")) ], ), ), ); } void executeExpensiveOperation() { debugPrint("Started"); int count = 0; for(int i=0; i<1000000000; i++){ count++; } debugPrint("Ended $count"); } } |
executeExpensiveOperation : – method having for loop running for million times which requires long time to complete. This is example is for heavy task this can be the image processing or huge volume of data handling in real world.
Executing Task
Execute the task by clicking on the elevated button
when we are clicking on the button heavy method start execution the user interface freezes for few seconds until the method complete its execution, because the main thread is busy to execute the task.
Increasing heavy task by 10 times
Increase the Operation 10 Times More
Now we have increase the operation 10 times more if we add one more zero in for loop termination condition. click on button again the UI will freeze for more than 10 seconds as show in above video, This will make bad user experience in real world application.
After adding isolates
To Solve UI freeze issue with the help of isolates we have to add below code inside on-pressed method of elevated button as shown below.
1 2 3 4 5 6 7 8 9 10 |
ElevatedButton(onPressed: () async { final receivePort = ReceivePort(); await Isolate.spawn(executeExpensiveOperation, receivePort.sendPort); receivePort.listen((message) { print("message --> $message"); }); } |
In above code two isolates are independently executes and complete the task, these isolates are communicate with each other by sending messages and values through ports (ReceivePort and SendPort).
ReceivePort :- In main isolates It listen for incoming messages and values from the new isolate.
SendPort :- To send a message from the new isolate back to the main isolate, use SendPort
object that was passed from the main isolate to the new isolate
Isolate.spawn() :- To create new Isolate using spawn method. spawn method required two parameters –
- first parameter is the method that want to execute with the help of new isolate.
- second parameter is the argument that is passed to the method.
listen() :- receivePort object provide method to listen for incoming message from new isolate and execute a callback function when receiving message.
Method to execute with New isolate
New isolate execute below method and receive a parameter as sendPort for sending the value to main isolate after completion.
1 2 3 4 5 6 7 8 9 10 |
void executeExpensiveOperation(SendPort sendPort) { debugPrint("Started"); int count = 0; for(int i=0; i<1000000000; i++){ count++; } debugPrint("Ended $count"); sendPort.send(count); } |
With Isolates
Solve UI freeze issue with the help of isolates.
Without Isolates
Conclusion
Thanks for reading this article ❤️
I hope this blog will help you to learn about how to use multithreading in flutter using isolates and you will be able to implement it. For more updates, make sure to keep following Mobikul Blogs to learn more about flutter and android.
Happy Learning ✍️
Reference
https://blog.logrocket.com/multithreading-flutter-using-dart-isolates/