Social Authentication like- Facebook, Google, Twitter, and Linkedin in the mobile apps is the most commonly used feature now a days . We can sign up and sign in to the app using the details of our social sites/app.
In this article, we are going to explore how we can integrate Google sign-in flutter.
You may also check our flutter app development services
So, let’s start.
First, we need to create an account and set up our project on Firebase.
We are assuming that you have set up your project on Firebase and are ready to move further in fluter code.
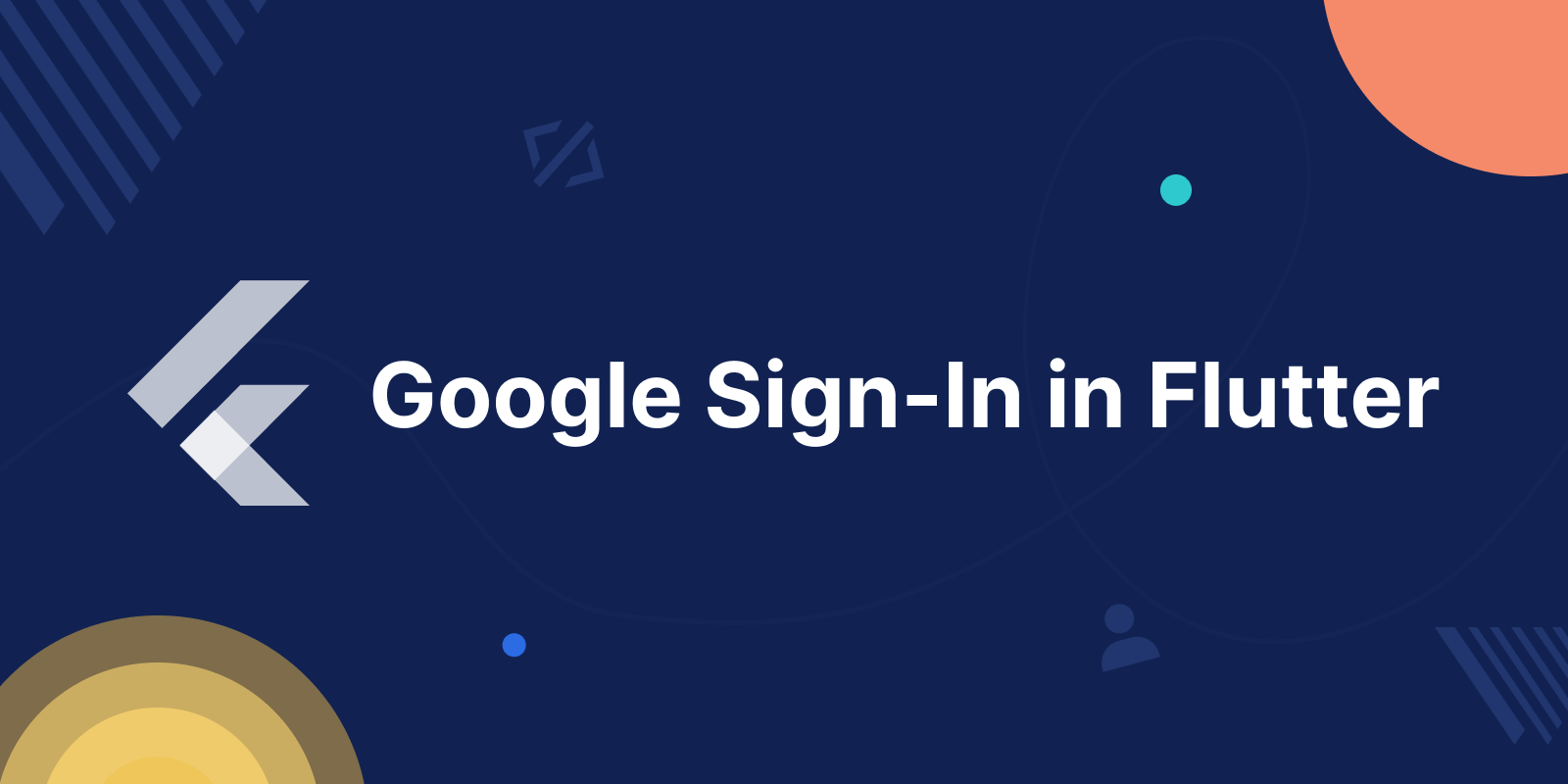
Android Integration:
Register your Android application in Firebase from the link.
Enable the OAuth APIs that you want, using the Google Cloud Platform API manager.
Note: Please fill in all required fields in the console for the OAuth consent screen. Otherwise, you might get APIException errors.
Download google-services.json file and add to the module (app-level) root directory of your app inside the Android folder.
IOS Integration:
Register your iOS application in Firebase from the link.
Download GoogleService-Info.plist file, and add in Runner target.
Add the below code in [your_project_name]/ios/Runner/Info.plist
file.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
<key>CFBundleURLTypes</key> <array> <dict> <key>CFBundleTypeRole</key> <string>Editor</string> <key>CFBundleURLSchemes</key> <array> <em><!-- TODO Replace this value: --></em> <em><!-- Copied from GoogleService-Info.plist key REVERSED_CLIENT_ID --></em> <string>com.googleusercontent.apps.861823949799-vc35cprkp249096uujjn0vvnmcvjppkn</string> </array> </dict> </array> |
Dependencies:
Add the below dependencies in the Pubspec file of your project
1 2 |
firebase_auth: ^0.16.0 google_sign_in: ^6.2.1 |
Import
1 2 3 |
import 'package:firebase_auth/firebase_auth.dart'; import 'package:firebase_core/firebase_core.dart'; import 'package:google_sign_in/google_sign_in.dart'; |
Example
Let’s take an example.
In this example, we have taken two screens and files.
Note: In this example, we are only handling the google login button event.
- Main.dart: In this file, we added a Google button. On the button click event, Google authentication will start.
- HomePage.dart: After successful authentication, we will be redirected to the Home screen.
Account details will be displayed on this screen.
main.dart
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 |
import 'package:firebase_auth/firebase_auth.dart'; import 'package:firebase_core/firebase_core.dart'; import 'package:flutter/material.dart'; import 'package:font_awesome_flutter/font_awesome_flutter.dart'; import 'package:google_sign_in/google_sign_in.dart'; import 'HomePage.dart'; Future<void> main() async { WidgetsFlutterBinding.ensureInitialized(); runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({ Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar(title: const Text("Google Login In Flutter")), body: _GoogleSignIn(key: null,), ), ); } } class _GoogleSignIn extends StatefulWidget { _GoogleSignIn({ Key? key}) : super(key: key); @override _GoogleSignInState createState() => _GoogleSignInState(); } class _GoogleSignInState extends State<_GoogleSignIn> { bool isLoading = false; @override Widget build(BuildContext context) { return Padding( padding: const EdgeInsets.all(10), child: ListView( children: <Widget>[ Container( alignment: Alignment.center, padding: const EdgeInsets.all(10), child: const Text( 'Webkul', style: TextStyle( color: Colors.blue, fontWeight: FontWeight.w500, fontSize: 30), )), Container( padding: const EdgeInsets.all(10), child: TextField( decoration: const InputDecoration( border: OutlineInputBorder(), labelText: 'User Name', ), ), ), Container( padding: const EdgeInsets.fromLTRB(10, 10, 10, 0), child: TextField( obscureText: true, decoration: const InputDecoration( border: OutlineInputBorder(), labelText: 'Password', ), ), ), TextButton( onPressed: () { //forgot password screen }, child: const Text('Forgot Password',), ), Container( height: 50, padding: const EdgeInsets.fromLTRB(10, 0, 10, 0), child: ElevatedButton( child: const Text('Login'), onPressed: () { }, ) ), Container( alignment: Alignment.center, padding: const EdgeInsets.all(10), child: const Text( 'OR', style: TextStyle(fontSize: 20), )), Container( height: 50, padding: const EdgeInsets.fromLTRB(10, 0, 10, 0), child: ElevatedButton( child: const Text('CLICK TO LOGIN VIA GOOGLE',style: TextStyle(fontWeight: FontWeight.bold,),), onPressed: () async { await Firebase.initializeApp(); setState(() { isLoading = true; }); FirebaseService service = new FirebaseService(context); try { await service.signInwithGoogle(); } catch (e) { if (e is FirebaseAuthException) { showErrorMessage(e.message??""); } } setState(() { isLoading = false; }); }, ) ), ], )); } void showErrorMessage(String message) { showDialog( context: context, builder: (BuildContext context) { return AlertDialog( title: Text("Error"), content: Text(message), actions: [ TextButton( child: Text("Ok"), onPressed: () { Navigator.of(context).pop(); }, ) ], ); }); } } class FirebaseService { final BuildContext parentContext; FirebaseService(this.parentContext); final FirebaseAuth _auth = FirebaseAuth.instance; final GoogleSignIn _googleSignIn = GoogleSignIn(); Future<String?> signInwithGoogle() async { try { print(" authentication start"); final GoogleSignInAccount? googleSignInAccount = await _googleSignIn.signIn(); final GoogleSignInAuthentication? googleSignInAuthentication = await googleSignInAccount?.authentication; final AuthCredential credential = GoogleAuthProvider.credential( accessToken: googleSignInAuthentication?.accessToken, idToken: googleSignInAuthentication?.idToken, ); UserCredential result = await _auth.signInWithCredential(credential); User? user = result.user; if (user != null) { print(" complete "+ user.photoURL!); } else { print("user Empty"); } Navigator.push( parentContext, MaterialPageRoute( builder: (context) => HomePage( name: user?.displayName, image: user?.photoURL, ), ), ); } on FirebaseAuthException catch (e) { print("auth error " + e.message!); throw e; } } } |
HomePage.dart
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 |
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; class HomePage extends StatelessWidget { const HomePage({ Key? key, this.name, this.image}) : super(key: key); final name; final image; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Welcome Screen "), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: [ Container( alignment: Alignment.center, padding: const EdgeInsets.all(10), child: const Text( 'Welcome', style: TextStyle( color: Colors.blue, fontWeight: FontWeight.w500, fontSize: 30), )), Padding( padding: const EdgeInsets.all(8.0), child: Container( alignment: Alignment.center, padding: const EdgeInsets.all(10), child: const Text( 'YOU HAVE SUCCESSFULLY LOGIN VIA GOOGLE ACCOUNT', textAlign: TextAlign.center , style: TextStyle( color: Color(0xFF112252), fontWeight: FontWeight.w500, fontSize: 18), )), ), Padding( padding: const EdgeInsets.all(8.0), child: Text("User name: "+name, style: TextStyle( color: Color(0xFF112252), fontWeight: FontWeight.w500, fontSize: 14),), ), Padding( padding: const EdgeInsets.all(8.0), child: Text("Profile Image: ",style: TextStyle( color: Color(0xFF112252), fontWeight: FontWeight.w500, fontSize: 14))), Padding( padding: const EdgeInsets.all(8.0), child: Container( alignment: Alignment.center, padding: const EdgeInsets.all(10), child: Image.network(image)), ), ], )), ); } } |
Now, run the code and check the result.
Result
Android App Result :
IOS App Result :
Conclusion :
In this blog, we have checked that how we can add Google authentication in the Flutter Apps.
For more information about social authentication please follow the Link.
Thanks For Reading…