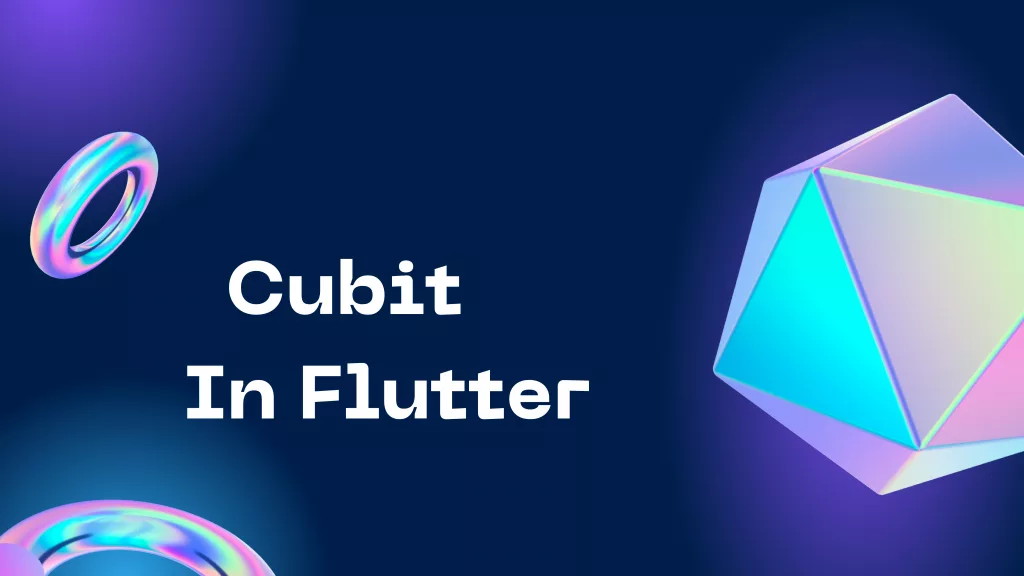
State management is a critical aspect of building robust and responsive Flutter applications. If you’re looking for an effective and efficient way to handle state in your Flutter projects, Cubit might be the solution you’ve been searching for.
In this comprehensive guide, we’ll take a deep dive into using the Cubit state management package to master state management in Flutter.
Introduction to Cubit
Cubit is a lightweight and powerful state management package designed for Flutter. It follows the BLoC (Business Logic Component) pattern and provides a structured way to handle the application’s state.
Cubit offers a clear separation of concerns, making your code more organized and maintainable. It’s also highly testable, which is crucial for building reliable applications.
Why Choose Cubit?
Before we dive into the details, let’s understand why you might want to use Cubit for state management and why Cubit is gaining popularity among Flutter developers:
- Simplicity: Cubit provides a straightforward and intuitive way to manage the state in your Flutter app. It’s easy to learn and use, making it suitable for both beginners and experienced developers.
- Testability: Cubit encourages test-driven development, making it easier to write unit tests for your app’s business logic. This ensures the reliability and stability of your code.
- Separation of Concerns: Cubit enforces a clear separation between the UI and business logic, promoting good coding practices and maintainability.
You may also check our Flutter app development company page.
Setting Up Cubit
To start using Cubit in your Flutter project, follow these steps:
Step 1: Create a New Flutter Project
1 |
flutter create your_project_name |
Step 2: Add the Cubit Dependency
Open your project’s “pubspec.yaml” file and add the Cubit dependency :
1 |
bloc: ^7.0.0 |
Run flutter pub get to fetch the dependencies.
Step 3: Create a Cubit
Now, you can create a Cubit. Cubits have two main components: states and events.
State: Represents the current state of your application.
Event: Represents an action or event that triggers a state change.
Create a Cubit class by extending the Cubit class and defining your states and events:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
import 'package:bloc/bloc.dart'; // Define states enum CounterState { initial, increased } // Define events class CounterEvent {} class CounterCubit extends Cubit<CounterState> { CounterCubit() : super(CounterState.initial); void increment() { emit(CounterState.increased); } } |
Step 4: Use Cubit in Your Widget Now that you have your Cubit, you can use it in your Flutter widgets.
Here’s an example of how to use the CounterCubit in a simple counter app:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
import 'package:flutter/material.dart'; import 'package:flutter_bloc/flutter_bloc.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: BlocProvider( create: (context) => CounterCubit(), child: CounterScreen(), ), ); } } class CounterScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Cubit Counter')), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ BlocBuilder<CounterCubit, CounterState>( builder: (context, state) { return Text( state == CounterState.increased ? 'Counter Increased!' : 'Initial State', style: TextStyle(fontSize: 24), ); }, ), SizedBox(height: 20), ElevatedButton( onPressed: () { context.read<CounterCubit>().increment(); }, child: Text('Increment Counter'), ), ], ), ), ); } } |
In this example, the BlocProvider widget provides the CounterCubit to the CounterScreen widget, and we use BlocBuilder to rebuild the UI when the state changes.
That’s it! You’ve successfully set up Cubit in your Flutter app.🥳🥳
Conclusion
In this guide, we’ve walked through the fundamentals of Cubit, from creating a Cubit class to integrating it into your widgets.
You can also check the Flutter official site for more information regarding Cubit.