In this article, we are going to learn about the Callable classes in Dart.
Read more about our Flutter app development services.
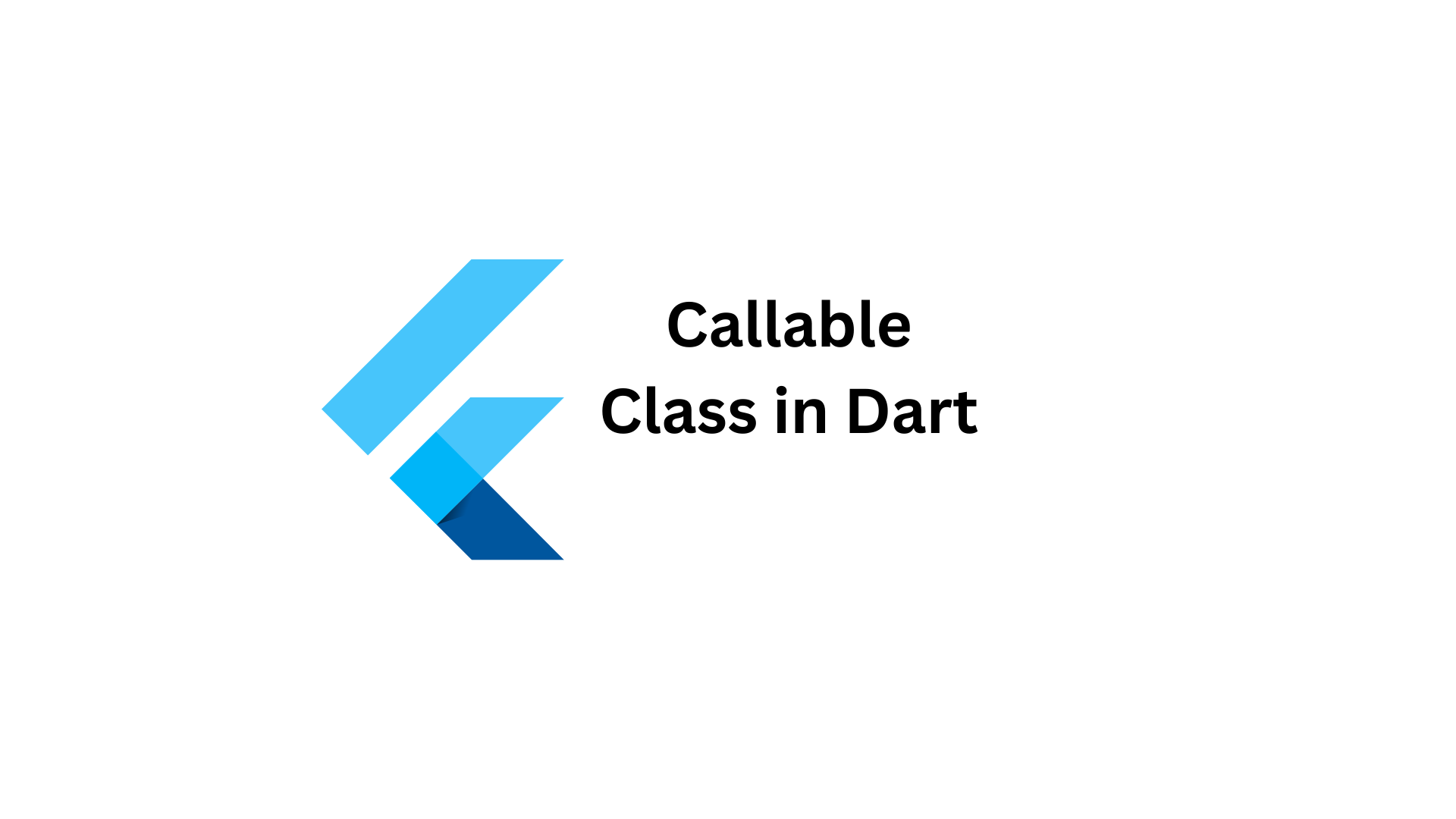
Introduction- Callable Classes
As the name “Callable” points out for calling something. Dart facilitates us to call the class instances like a function with the help of the Call() function.
Basically, If we want to call a class instance as a function then we need to implement the call() function in it.
The call () method supports the same functionality as normal functions such as parameters and return types.
Syntax
1 2 3 4 5 6 7 8 |
class your_class_name { return_type call ( parameters ) { ... // function code } } |
Implementation
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
class myCallableClassExample { String call(String a, String b, String c) => ' $a $b $c !'; // call method which to make create the class callable } void main() { // Creating instance of class var callableClassInstance = myCallableClassExample(); var callableClassReturn = callableClassInstance('Hello', 'Team', 'Mobikul'); print(callableClassReturn); } |
Result:
Callable function example for int return type
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
class myCallableClassExample { int call (int a,int b, int c)=>a+b+c;// call method make create the class callable } void main() { var callableClassInstance = myCallableClassExample();// Creating instance of class var callableClassReturn = callableClassInstance(5, 10, 15); print(callableClassReturn); } |
Result:
Till now, we know about call function and how to implement them to make a class callable.
Now A question arises, Can we create multiple call functions for a class?
The answer is NO. Dart doesn’t allow us to create multiple call()
functions for a class.
Let’s check it with an example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
class myCallableClassExample { int call (int a,int b, int c)=>a+b+c;// Fisrt call method make create the class callable int call (int a,int b, int c)=>a+b+c;// Second call method } void main() { var callableClassInstance = myCallableClassExample();// Creating instance of class var callableClassReturn = callableClassInstance(5, 10, 15); print(callableClassReturn); } |
Result:
Conclusion :
In this article, we have checked about callable classes and call() function.
Thanks for Reading.