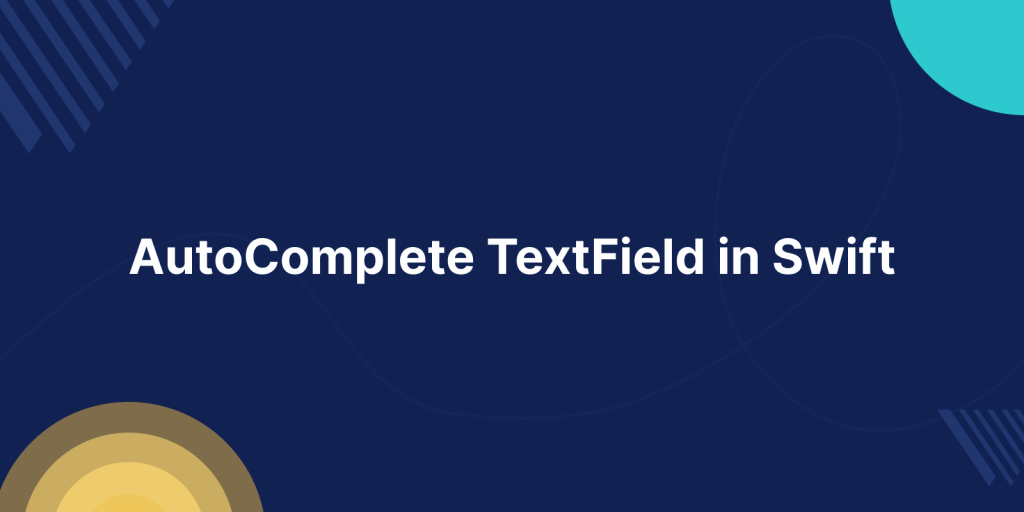
UITextField can be used to add an autocomplete feature, like in Safari, to your iOS apps to suggest a word based on the characters your user types.
AutoComplete text field is a feature that shows completion suggestions automatically while the user is typing.
Please follow below steps to implement autocomplete TextField example in your iOS app.
Step 1: Add a TextField in your UIViewController and make an outlet of textField in ViewController class. Also set delegate of your text field to self in viewdidload().
Step 2: Declare an array of suggestions element to show in textField.
1 |
let suggestionsArray = [ "black", "blue", "green", "yellow", "orange", "purple" ] |
Step 3: Now, use UITextField delegate “shouldChangeCharactersIn” function to handle user input in textField.
1 2 3 |
func textField(_ textField: UITextField, shouldChangeCharactersIn range: NSRange, replacementString string: String) -> Bool { return !autoCompleteText( in : textField, using: string, suggestionsArray: suggestionsArray) } |
Step 4: TextField delegate method textField(_:shouldChangeCharactersIn:replacementString:) calls “autoCompleteText” method which searches the suggestion list for the first entry with the prefix matching the user input. And updates the text value with the identified suggestion. “autoCompleteText” method is defined as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
func autoCompleteText( in textField: UITextField, using string: String, suggestionsArray: [String]) -> Bool { if !string.isEmpty, let selectedTextRange = textField.selectedTextRange, selectedTextRange.end == textField.endOfDocument, let prefixRange = textField.textRange(from: textField.beginningOfDocument, to: selectedTextRange.start), let text = textField.text( in : prefixRange) { let prefix = text + string let matches = suggestionsArray.filter { $0.hasPrefix(prefix) } if (matches.count > 0) { textField.text = matches[0] if let start = textField.position(from: textField.beginningOfDocument, offset: prefix.count) { textField.selectedTextRange = textField.textRange(from: start, to: textField.endOfDocument) return true } } } return false } |
The method returns true if the match was found otherwise returns false. The delegate method “shouldChangeCharactersIn” returns the inverse of this value so the text field will continue to process keystrokes when a match is not found.
Step 5 : Finally, the controller implements the delegate’s textFieldShouldReturn(_:)
method to “confirm” the suggestion:
1 2 3 4 |
func textFieldShouldReturn(_ textField: UITextField) -> Bool { textField.resignFirstResponder() return true } |
Step 6: Now, compile your project and you can see the results.
Hope this article helps you.
For any queries, please feel free to add a comment in the comments section.